Apple Pay and in-app purchases
The good news: now with Flitt you can integrate Apple Pay into your mobile application natively, without any redirects.
Businesses using Apple Pay with Flitt may see these benefits:
- Faster transactions: On average transactions are processed in less than 2 seconds.
- No 3D-Secure authentication: only Face ID and Touch ID authentication will not distract payer from the checkout process.
- Better conversion: more than 97% of approved transactions.
- Low fees: no additional fees on wallet transactions.
- Better fraud defence: Apple Pay’s advanced security features protect businesses from potential financial losses. The chargeback rate for Apple Pay is significantly lower due to such technologies as card tokenization, a dedicated chip known as the Secure Element and Apple Pay’s algorithms to detect potential fraud and prevent unauthorized transactions.
- Subscriptions and recurring payments: after initial payment is done by the client, you can further charge regular payments without client presence.
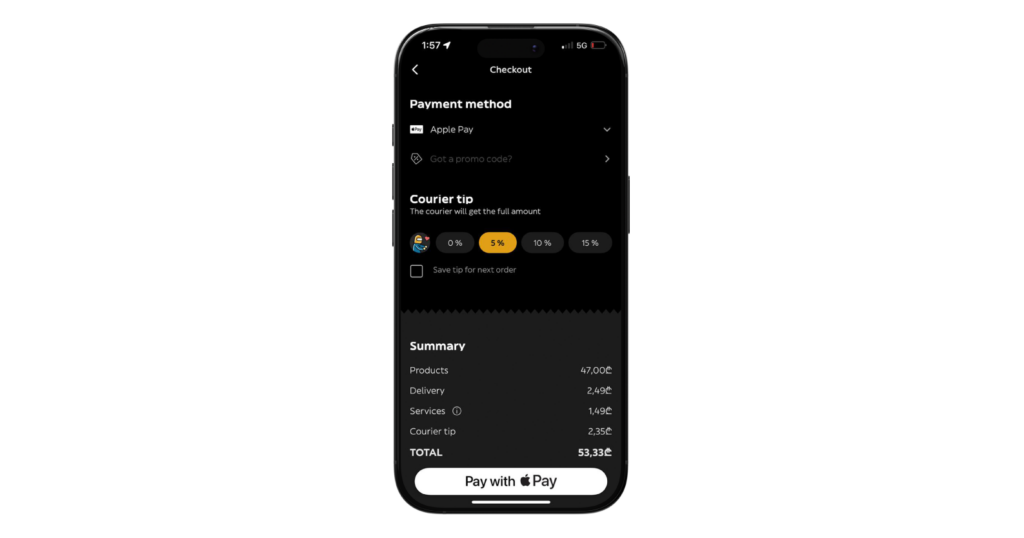
Before we start talking about Apple Pay integration, let’s pay attention to one Apple restriction:
It is important to understand that Apple Pay with Flitt is not a substitute for in-app purchases. In-app purchases do not go through Flitt and are subject to Apple’s 30% fee. While Flitt and the fees process Apple Pay purchases are significantly lower (less than 2%).
However, Apple Pay in a mobile application with Flitt is available only for physical goods or services as well as donations, subscriptions, club memberships, hotel reservations, and tickets for events. Apple’s developer documentation clearly suggests that in-app purchases are for virtual goods and digital content. This is something you should clearly understand before deciding which type of payment method to integrate because otherwise you might have your app rejected and you will lose a lot of time.
If you still need ApplePay with Flitt for digital/virtual goods, there are two options:
- redirect customers from the mobile application to Apple Pay on the web with redirect
- embed the ApplePay button in webview with JavaScript embeded Flitt integration.
So, to start the integration without redirect and webview (native ApplePay button), you’ll have to go through these steps:
- Create Apple Pay developer account
- Register Apple Merchant ID
- Create new Apple pay certificates
- Setup Xcode
- Create payment token on the backend
- Create a payment button in the mobile application
- Process result from Flitt
- Initiate recurring payment if needed
Create Apple Pay developer account
So, let’s start with the Apple Pay developer account. If you don’t have it, you will need to enrol ($99/yr) here. It will be required for development and application approval.
Register Apple Merchant ID
The next step is to register an Apple Merchant ID. Follow the instructions to register merchant ID at the Apple Developer site. For step-by-step instructions, you can also refer to the Flit documentation.
As a result, you will obtain an Apple Merchant ID like this: merchant.flitt.com.{{your_app_bundle_id}}.
Create new Apple Pay certificates
For further Apple Pay payment processing, Flit will need additional certificates to decrypt payloads and communicate with Apple API.
Create new Apple Pay certificates ****and send them to Flitt support.
There should be
- either four DER files merchant_id.cer, merchant_id.key, apple_pay.cer, apple_pay.key
- either two PEM files merchant_id.pem and apple_pay.pem
- either two P12 merchant_id.p12 and apple_pay.p12
File names can slightly differ, but extensions must be strictly the same as specified.
Setup Xcode
Go to XCode -> Target -> Capabilities -> ApplePay -> Merchant IDS and setup your registered Apple Merchant ID merchant.flitt.com.{{your_app_bundle_id}}.
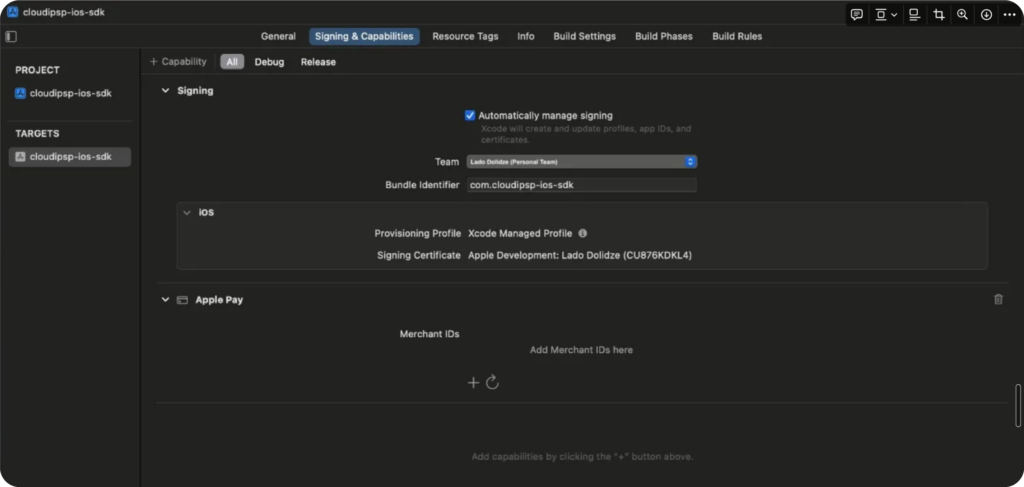
Create payment token on the backend
The best practice for mobile application architecture is to create a payment on your backend and further manage its statuses only on backend. This logic is secure and will mitigate the risks when some payment data can be substituted by unauthorized client actions on frontend .
To initiate payment on the backend and obtain a payment token, follow Flitt documentation page.
Create payment at your server:
curl -i -X POST \\
-H "Content-Type:application/json" \\
-d \\
'{
"request": {
"server_callback_url": "<http://myshop/callback/>",
"order_id": "TestOrder_JSSDK_v2",
"currency": "GEL",
"merchant_id": 1549901,
"order_desc": "Test payment",
"lifetime" : 999999,
"amount": 1000,
"signature": "91ea7da493a8367410fe3d7f877fb5e0ed666490"
}
}' \\
'<https://pay.flitt.com/api/checkout/token>'
Receive payment token:
{
"response":{
"response_status":"success",
"token":"b3c178ad84446ef36eaab365b1e12e6987e9b3d9"
}
}
Store payment token in your database or in other storage along with other payment data and payment unique ID order_id
. Pay attention that to get a new payment token you will need to generate a unique order_id
each time. But you also can reuse existing payment token if the payment amount has not changed.
Create a payment button in the mobile application
Apple Pay comes with specific guidelines for the theme of the distinct Buy button: which should be dark or light according to the background colour around it as an element, as well as for the button size, and the position of the button in the context of the environment it is being included.
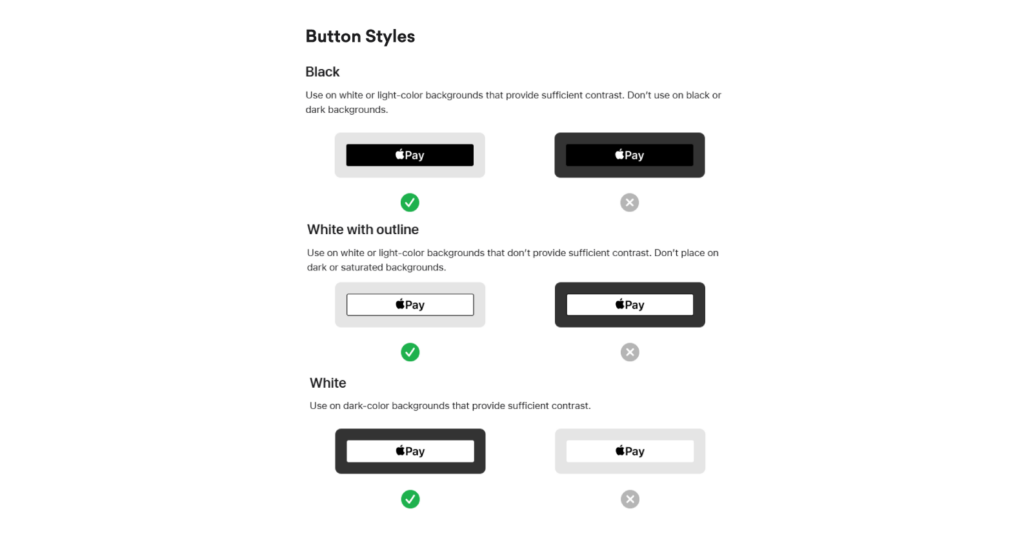
Apple Pay in mobile application can be integrated with Flitt SDK depending on the programming language or framework:
Before displaying the Apple Pay button in your mobile application, check if the user’s device supports Apple Pay and that they have a card added to their wallet with the method:
- (IBAction)applePayClicked:(id)sender {
if ([PSCloudipspApi supportsApplePay]) {
If ApplePay is supported, process payment with the token:
- (void)applePayWithToken:(NSString *)token
andDelegate:(id<PSApplePayCallbackDelegate>)delegate {
Process result from Flitt
Process the result of Apple Pay payment with:
- callback on frontend:
@protocol PSPayCallbackDelegate <NSObject>
- (void)onPaidProcess:(PSReceipt *)receipt;
- (void)onPaidFailure:(NSError *)error;
- (void)onWaitConfirm;
- server-to-server callback on the backend: see Receiving Callbacks
Your application should trust only the callback which is signed with a signature. Signature successful validation is crucial before approval of the order in your application business logic.
Testing
You can use Apple Pay sandbox and Flitt test cards to check if you implemented it correctly.
Pay attention that while your application is not in production mode, Flitt merchant settings must be also in test mode. Please contact our support team to align these settings.
Subscriptions and recurring payments
Now let’s speak on how to organize regular payments without customer interaction.
There are two main types of such payments:
- subscription – when a payment calendar is stored and managed on Flitt side
- recurring – when a payment calendar is stored on your application side
The difference is obvious: subscription is easier to implement, but recurring is a more flexible and advanced solution.
For subscriptions, you don’t need to do much more than to pass several parameters describing periodicity to Flitt SDK:
recurring_data: {
every: 1,
amount: 1000,
period: "month",
end_time: "2026-11-14",
start_time: "2019-11-11"
}
For recurring payments, you need previously to obtain a recurring token rectoken
for each client.
Please refer to the article Saving cards in our documentation on how to get rectoken
.
rectoken
is the link to the client’s Apple Pay payment data which Flitt securely stores encrypted. So you don’t need to deal with the security mechanics for this process, just save rectoken
in your client’s payment calendar. When it is time to charge the client with the next payment, call method Create recurring order with saved card to initiate Apple Pay payment without client interaction.
Summary
Connecting Apple Pay in the application can be a pain point for developers. While Apple Pay documentation is clear and full enough, the integration process is complicated and has many requirements and use cases.
That’s why Flitt’s team is happy to share with you our expertise in implementing Apple Pay.
Flitt Apple Pay mobile SDK saves you a huge amount of resources and boosts up your business revenue growth.
Feel free to ask questions, comment, and share. We would be happy to help you with the development of your application!